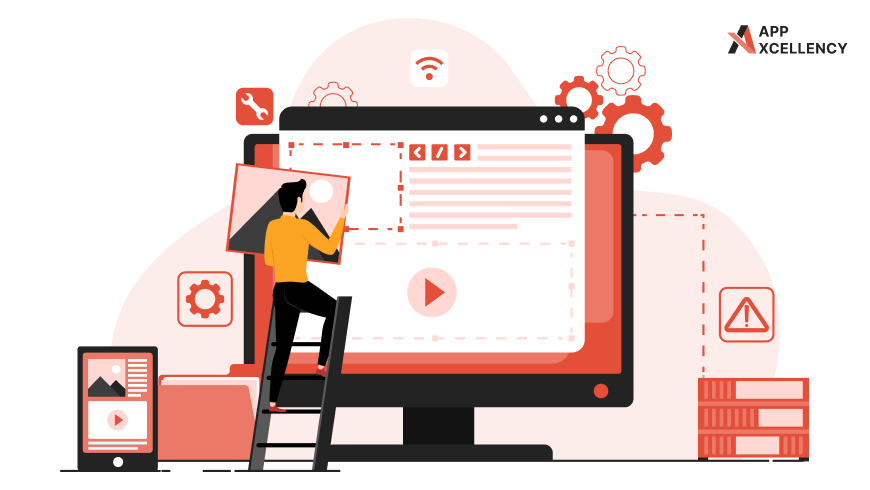
Have you ever felt limited by what WordPress offers out of the box? Well, that’s where plugins come in!
WordPress plugins are like mini-programs that you can simply install on your WordPress website to add new features and functionalities.
In other words, they are similar to apps for your smartphone, but for your website. They allow you to easily customize your site without needing to be a coding expert.
Now, coming to plugins, tons of great ones are already available on the market. But what if you have a specific requirement that none of the plugins address?
This is where custom plugins come into the picture.
Building your own plugin can create a tailored solution that perfectly fits your website’s unique requirements.
In this blog post, we will walk you through a tutorial that is designed for beginners who have a basic understanding of PHP.
The tutorial consists of steps to create your first WordPress plugin, giving you the power to build custom functionalities and extend your website’s potential.
Getting Started
Before we dive into the code, let’s get your development fundamentals right!
1. Setting Up Your Tools:
- Text Editor or IDE: This is where you will write your plugin code. Popular free options include Visual Studio Code, Sublime Text, or Atom. Choose one you feel comfortable with!
- Local WordPress Installation: This allows you to test your plugin without affecting your live website. There are several ways to achieve this:
- Local development tools: Tools like Local by Flywheel or DesktopServer offer user-friendly interfaces to set up a local WordPress environment.
- Manual setup (advanced): You can manually install a LAMP/LEMP stack (Linux, Apache/Nginx, MySQL/MariaDB, PHP) on your computer and then install WordPress.
2. Understanding Plugin Structure:
Now that you have your tools ready, let’s explore how WordPress plugins are organized:
- Plugin Folder: Each plugin has its own dedicated folder within the WordPress /wp-content/plugins directory. This folder will hold all the files that make up your plugin.
- Main Plugin File (plugin-name.php): This is the heart of your plugin. It contains essential information like the plugin name, description, and the code that defines its functionality.
3. Building Your First Plugin: Hello World!
Let’s create a simple “Hello World” plugin to get familiar with the basic structure. Here’s a step-by-step walkthrough:
- Create a new folder within your WordPress plugins directory (e.g., hello-world).
- Inside this folder, create a file named hello-world.php. This will be your main plugin file.
- Open hello-world.php in your text editor and paste the following code:
PHP <?php /** * Plugin Name: Hello World! * Plugin URI: # (This will be filled later) * Description: A simple plugin to test the development environment. * Version: 1.0.0 * Author: Your Name * Author URI: # (This will be filled later) * License: GPLv2 or later */ // Display a message on the admin panel add_action( ‘admin_notices’, ‘hello_world_notice’ ); function hello_world_notice() { echo ‘<div class=”notice notice-success”> <p>Hello World! This is your first WordPress plugin.</p> </div>’; } |
4. Save the file:
Explanation:
- This code defines the plugin header with essential information like name, description, author, etc.
- It then uses the add_action function to hook into the admin_notices action, which runs on the WordPress admin dashboard.
- The hello_world_notice function creates a simple success message displayed on the admin panel, confirming your plugin is working.
5. Activating Your Plugin:
- In your WordPress admin panel, navigate to Plugins > Add New.
- Click “Upload Plugin” and select the hello-world folder you created.
- Click “Install Now” and then activate the plugin.
Now, visit your WordPress admin dashboard. You should see the “Hello World!” message displayed, confirming your first successful plugin creation!
Core Plugin Functionality
Now that you’ve built your first basic plugin, let’s delve into some core functionalities that make WordPress plugins truly powerful.
1. Plugin Header: The Information Hub
The plugin header sits at the top of your main plugin file (plugin-name.php) and acts like an identity card for your plugin. It provides essential information about WordPress that’s displayed in various places like the plugin list and admin screens.
Here’s a breakdown of the required and optional fields:
- Required Fields:
- Plugin Name: This is the name users will see in the WordPress admin panel.
- Description: A brief explanation of what your plugin does. Keep it informative and engaging!
- Author: Your name or your development company’s name.
- Version: The current version number of your plugin (e.g., 1.0.0). Update this as you add new features.
- Optional Fields:
- Plugin URI: A link to your website or the plugin’s homepage, if applicable.
- Author URI: A link to your website or profile page.
- License: Specify the license under which you are distributing your plugin (e.g., GPLv2 or later).
Here’s an example header snippet to illustrate these points:
PHP <?php/** * Plugin Name: My Custom Functionality Plugin * Plugin URI: https://yourwebsite.com/my-plugin * Description: This plugin adds a custom button with a specific action to your WordPress admin panel. * Version: 1.0.1 * Author: Your Name * Author URI: https://yourprofile.com * License: GPLv2 or later */ |
2. Hooks and Filters: Interacting with WordPress
Hooks and filters are the magic ingredients that allow your plugins to interact with different parts of WordPress and modify its behavior.
- Hooks: Think of hooks as designated points in WordPress code where you can insert your own custom functionality. You can “hook into” these points using functions like add_action or add_filter to execute your code at specific moments.
- Filters: Filters allow you to modify existing data within WordPress. You use functions like add_filter to intercept data as it’s processed and potentially change it before it’s used by WordPress.
Here are some basic examples:
- Adding a custom button to the admin panel with add_action:
PHPadd_action( ‘admin_menu’, ‘my_custom_button’ ); function my_custom_button() { // Code to create and display your custom button} |
- Modifying the title of a post using add_filter:
PHPadd_filter( ‘the_title’, ‘modify_post_title’ ); function modify_post_title( $title ) { // Code to modify the post title (e.g., prepend or append text) return $modified_title;} |
These are just basic examples, but hooks and filters offer a vast range of possibilities for customizing WordPress functionality through your plugins.
Building Your Plugin: Adding a Custom Button with Action
Now, let’s put your newfound knowledge into practice! We’ll build a plugin that adds a custom button to your WordPress admin panel, allowing you to trigger a specific action when clicked.
1. Desired Functionality:
Imagine you want to easily clear your website cache from the admin panel. Our plugin will add a custom button labeled “Clear Cache” that, when clicked, will execute a function to clear the cache.
2. Code Walkthrough:
- Adding the functionality: Open your main plugin file (plugin-name.php).
- Hooking into the admin menu: We’ll use the add_action function to hook into the admin_menu action, which fires when the admin menu is built. This ensures our button is added to the appropriate location.
PHPadd_action( ‘admin_menu’, ‘my_custom_button’ ); function my_custom_button() { // Code to create and display the button} |
3. Creating the button: Inside the my_custom_button function, we’ll use HTML code to create a button element with the desired label (“Clear Cache”). We’ll also define a unique ID for the button for further reference.
PHPfunction my_custom_button() { // Add a new top-level menu (optional, can be customized) add_menu_page( ‘My Custom Plugin’, ‘My Plugin’, ‘manage_options’, ‘my-plugin-settings’, ”, ”, 20 ); // Inside the menu page (optional, based on your menu choice) echo ‘<div class=”my-plugin-settings-container”>’; echo ‘<button id=”clear-cache-button”>Clear Cache</button>’; echo ‘</div>’;} |
4. Defining the button action: We’ll use JavaScript to capture the button click event and then call a function (e.g., clear_cache) to perform the desired action (clearing the cache).
PHPfunction my_custom_button() { // … (previous code) echo ‘<script> document.getElementById(“clear-cache-button”).addEventListener(“click”, function() { clear_cache(); // Call the function to clear cache }); </script>’;} |
Explanation:
- We’ve added the button creation code within an optional add_menu_page function. This creates a dedicated menu page for your plugin, where the button resides. You can customize this placement based on your needs.
- The button has a unique ID (clear-cache-button) for easy reference in the JavaScript code.
- The JavaScript code listens for clicks on the button and then calls the clear_cache function (which you’ll need to define later in your plugin code to handle the actual cache clearing logic).
3. Optional: Adding Settings Page
For more complex plugins, you might want to allow users to configure settings through a dedicated settings page. Here’s a brief overview:
- Creating the Settings Page: Use the add_menu_page function to create a new menu item for your plugin settings.
- Building the Form: Use HTML to create a form with input fields for your settings.
- Handling Form Submissions: Use the add_action function to hook into the form submission action and process the submitted data to update your plugin settings.
Note: This is a simplified explanation of creating a settings page. There are additional steps and security considerations involved in a full implementation.
By following these steps and adding the clear_cache function with your specific cache clearing logic, you’ll have a working plugin that demonstrates the power of hooks, buttons, and optional settings pages in WordPress plugin development.
Deploying and Testing Your Plugin
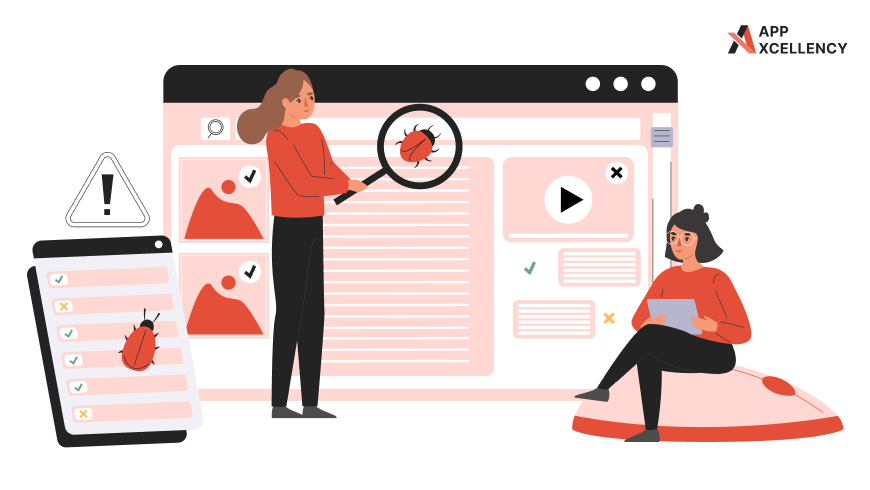
Congratulations, you’ve built your first custom plugin, it’s now time to get it running on your WordPress website! Here’s how to deploy and test your creation.
1. Packaging as a Zip File:
- Once you’ve completed your plugin code and ensured everything is functioning as intended, navigate to the folder containing your plugin files (e.g., hello-world or your custom button plugin folder).
- Use a file compression tool like WinRAR or 7-Zip to compress the entire folder into a zip archive.
2. Uploading and Activating the Plugin:
- Log in to your WordPress admin panel.
- Navigate to Plugins > Add New.
- Click “Upload Plugin” and select the zip file you just created containing your plugin folder.
- Click “Install Now” and then activate the plugin.
3. Testing Thoroughly:
- After activation, rigorously test your plugin’s functionality.
- In our example, click the “Clear Cache” button and verify if your cache clearing logic works as expected (you might need to test with a caching plugin installed).
- Think of various scenarios and edge cases to ensure your plugin behaves correctly under different conditions.
Tips:
- It’s highly recommended to test your plugin on a staging site (a development copy of your live website) before deploying it to your production site to avoid any potential issues on your live website.
- Always back up your website before activating a new plugin, just in case something unexpected happens.
By following these steps, you’ve successfully deployed and tested your first WordPress plugin! Now you can explore building more complex plugins that extend your website’s functionality and tailor it to your specific needs.
Final Thoughts
Yaay! You’ve taken your first steps into the exciting world of WordPress plugin development. In this tutorial, we’ve covered the essential concepts to get you started:
- Understanding the purpose and benefits of custom plugins.
- Setting up your development environment and exploring plugin structure.
- Learning about plugin headers and their importance.
- Grasping the power of hooks and filters for interacting with WordPress.
- Building a basic plugin with a custom button and action.
- Deploying and testing your plugin to ensure proper functionality.
This is just the beginning! There’s a vast world of possibilities when it comes to building WordPress plugins. Here are some resources to fuel your further learning:
- WordPress Plugin Handbook: https://developer.wordpress.org/plugins/intro/ – This comprehensive resource from WordPress itself dives deep into all aspects of plugin development, from basic concepts to advanced techniques.
- Online Tutorials: There are numerous online tutorials and courses available that offer step-by-step guidance on building various types of WordPress plugins. Search for topics that interest you and explore different learning styles.
Don’t be afraid to experiment and build more complex plugins as you gain confidence. Remember, the best way to learn is by doing! So, start brainstorming ideas for how you can leverage WordPress plugins to enhance your website and unleash your creativity.